ABOUT
PHP
In today's digital age, having a strong online presence is paramount. Achieving this requires a robust and versatile web development framework, and that's precisely where Core PHP comes into play. As the cornerstone of dynamic web solutions, Core PHP is a server-side scripting language that empowers developers to create websites and web applications that are visually captivating and functionally robust.
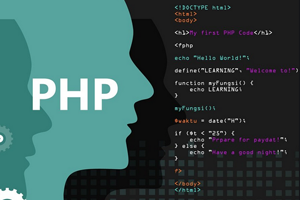
FEATURES
PHP
SYLLABUS
Introduction to PHP
Basic PHP Syntax
Functions
Arrays
Working with Forms
File Handling
Database connectivity
Object-Oriented Programming (OOP) in PHP
Session Management
Error Handling
Security
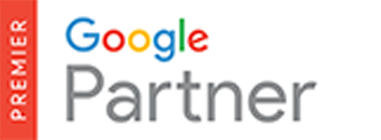
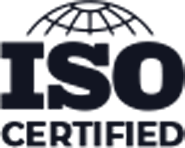
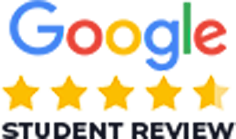
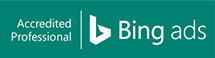
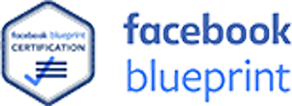